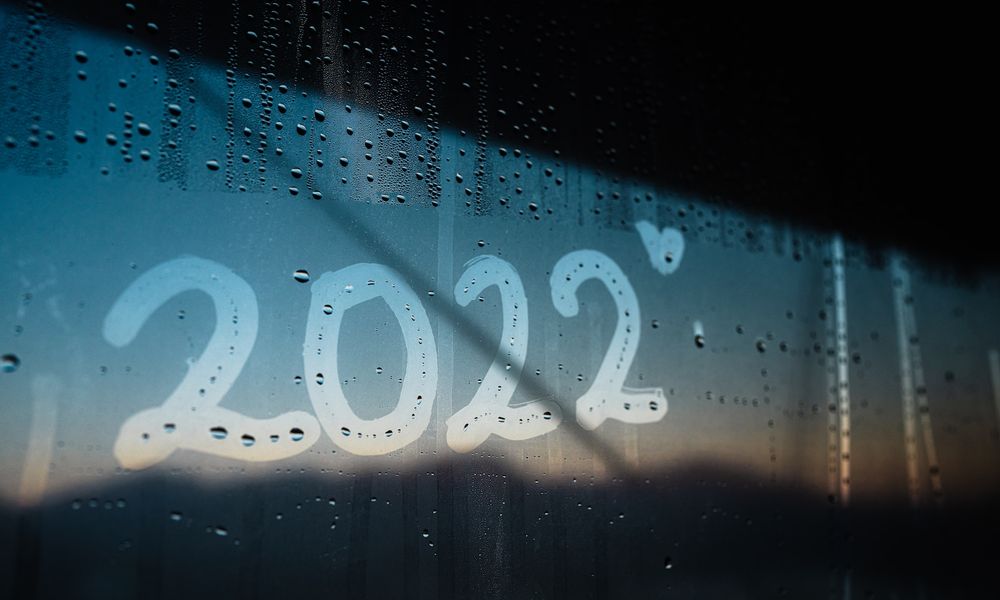
Due to the discussions and interests sparked by my previous article about ECMAScript 2023 (at least within my friends and colleagues 😃), I decided to write a follow up article to cover what was added to the language in the 2022 version.
Top level await
The await keyword can now be used at the top level of a module. This means that you can now use await outside of an async function. This is useful for top-level await in modules that are loaded asynchronously, such as modules loaded with dynamic import.
Example:
// Importing a module asynchronously
const module = await import("./module.js");
or
import { doSomethingAsyncronously } from "./module.js";
const result = await doSomethingAsyncronously();
.at() method for arrays
This method returns the element at the specified index. If the index is negative, the element is returned from the end of the array.
It’s a welcome addition to the language, as it makes it easier to get an element from an array without having to slice it first. It’s also far more readable.
Example:
const array = [1, 2, 3, 4, 5];
const element = array.at(2);
console.log(element); // 3
Error cause
The Error object now has a cause property that can be used to get the original error that caused the current error.
Example:
try {
// Some code that throws an error
} catch (error) {
throw new Error("Something went wrong", { cause: error }); // original error is now available in the cause property
}
Conclusion
In my personal opinion the 2022 version was even more exiting then the 2023 version. The top level await and the cause property for errors are both very useful additions to the language. The .at() it’s more a nice to have. But I really enjoy writing and reading concise code, so I’m really happy it was added to the language.
Until the next time, keep on coding!